The next release of .NET and Visual Studio include a ton of great new features and capabilities. With ASP.NET 4.5 you'll see a bunch of really nice improvements with both Web Forms and MVC - as well as in the core ASP.NET base foundation that both are built upon.
This post covers some of the work done to add built-in support for bundling and minification into ASP.NET - which makes it easy to improve the performance of applications. This feature can be used by all ASP.NET applications, including both ASP.NET MVC and ASP.NET Web Forms solutions.
Basics of Bundling and Minification
As more and more people use mobile devices to surf the web, it is becoming increasingly important that the websites and apps we build perform well with them. We’ve all tried loading sites on our smartphones – only to eventually give up in frustration as it loads slowly over a slow cellular network. If your site/app loads slowly like that, you are likely losing potential customers because of bad performance. Even with powerful desktop machines, the load time of your site and perceived performance can make an enormous customer perception.
Most websites today are made up of multiple JavaScript and CSS files to separate the concerns and keep the code base tight. While this is a good practice from a coding point of view, it often has some unfortunate consequences for the overall performance of the website. Multiple JavaScript and CSS files require multiple HTTP requests from a browser – which in turn can slow down the performance load time.
Simple Example
Below I’ve opened a local website in IE9 and recorded the network traffic using IE’s built-in F12 developer tools. As shown below, the website consists of 5 CSS and 4 JavaScript files which the browser has to download. Each file is currently requested separately by the browser and returned by the server, and the process can take a significant amount of time proportional to the number of files in question.
Bundling
ASP.NET is adding a feature that makes it easy to “bundle” or “combine” multiple CSS and JavaScript files into fewer HTTP requests. This causes the browser to request a lot fewer files and in turn reduces the time it takes to fetch them. Below is an updated version of the above sample that takes advantage of this new bundling functionality (making only one request for the JavaScript and one request for the CSS):
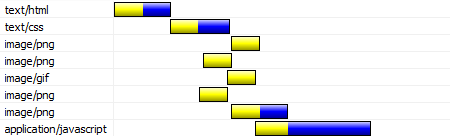
The browser now has to send fewer requests to the server. The content of the individual files have been bundled/combined into the same response, but the content of the files remains the same - so the overall file size is exactly the same as before the bundling. But notice how even on a local dev machine (where the network latency between the browser and server is minimal), the act of bundling the CSS and JavaScript files together still manages to reduce the overall page load time by almost 20%. Over a slow network the performance improvement would be even better.
Minification
The next release of ASP.NET is also adding a new feature that makes it easy to reduce or “minify” the download size of the content as well. This is a process that removes whitespace, comments and other unneeded characters from both CSS and JavaScript. The result is smaller files, which will download and load in a browser faster. The graph below shows the performance gain we are seeing when both bundling and minification are used together:
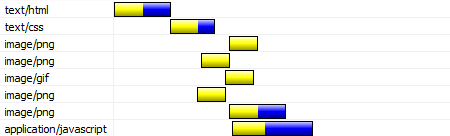
Even on my local dev box (where the network latency is minimal), we now have a 40% performance improvement from where we originally started. On slow networks (and especially with international customers), the gains would be even more significant.
Using Bundling and Minification inside ASP.NET
The upcoming release of ASP.NET makes it really easy to take advantage of bundling and minification within projects and see performance gains like in the scenario above. The way it does this allows you to avoid having to run custom tools as part of your build process – instead ASP.NET has added runtime support to perform the bundling/minification for you dynamically (caching the results to make sure perf is great). This enables a really clean development experience and makes it super easy to start to take advantage of these new features.
Let’s assume that we have a simple project that has 4 JavaScript files and 6 CSS files:
Bundling and Minifying the .css files
Let’s say you wanted to reference all of the stylesheets in the “Styles” folder above on a page. Today you’d have to add multiple CSS references to get all of them – which would translate into 6 separate HTTP requests:
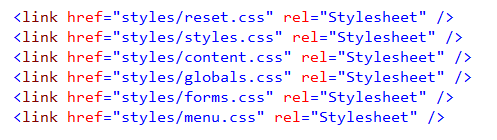
The new bundling/minification feature now allows you to instead bundle and minify all of the .css files in the Styles folder – simply by sending a URL request to the folder (in this case “styles”) with an appended “/css” path after it. For example:

This will cause ASP.NET to scan the directory, bundle and minify the .css files within it, and send back a single HTTP response with all of the CSS content to the browser.
You don’t need to run any tools or pre-processor to get this behavior. This enables you to cleanly separate your CSS into separate logical .css files and maintain a very clean development experience – while not taking a performance hit at runtime for doing so. The Visual Studio designer will also honor the new bundling/minification logic as well – so you’ll still get a WYSWIYG designer experience inside VS as well.
Bundling and Minifying the JavaScript files
Like the CSS approach above, if we wanted to bundle and minify all of our JavaScript into a single response we could send a URL request to the folder (in this case “scripts”) with an appended “/js” path after it:

This will cause ASP.NET to scan the directory, bundle and minify the .js files within it, and send back a single HTTP response with all of the JavaScript content to the browser. Again – no custom tools or builds steps were required in order to get this behavior. And it works with all browsers.
Ordering of Files within a Bundle
By default, when files are bundled by ASP.NET they are sorted alphabetically first, just like they are shown in Solution Explorer. Then they are automatically shifted around so that known libraries and their custom extensions such as jQuery, MooTools and Dojo are loaded before anything else. So the default order for the merged bundling of the Scripts folder as shown above will be:
- Jquery-1.6.2.js
- Jquery-ui.js
- Jquery.tools.js
- a.js
By default, CSS files are also sorted alphabetically and then shifted around so that
reset.css and
normalize.css (if they are there) will go before any other file. So the default sorting of the bundling of the Styles folder as shown above will be:
- reset.css
- content.css
- forms.css
- globals.css
- menu.css
- styles.css
The sorting is fully customizable, though, and can easily be changed to accommodate most use cases and any common naming pattern you prefer. The goal with the out of the box experience, though, is to have smart defaults that you can just use and be successful with.
Any number of directories/sub-directories supported
In the example above we just had a single “Scripts” and “Styles” folder for our application. This works for some application types (e.g. single page applications). Often, though, you’ll want to have multiple CSS/JS bundles within your application – for example: a “common” bundle that has core JS and CSS files that all pages use, and then page specific or section specific files that are not used globally.
You can use the bundling/minification support across any number of directories or sub-directories in your project – this makes it easy to structure your code so as to maximize the bunding/minification benefits. Each directory by default can be accessed as a separate URL addressable bundle.
Bundling/Minification Extensibility
ASP.NET’s bundling and minification support is built with extensibility in mind and every part of the process can be extended or replaced.
Custom Rules
In addition to enabling the out of the box - directory-based - bundling approach, ASP.NET also supports the ability to register custom bundles using a new programmatic API we are exposing.
The below code demonstrates how you can register a “customscript” bundle using code within an application’s Global.asax class. The API allows you to add/remove/filter files that go into the bundle on a very granular level:
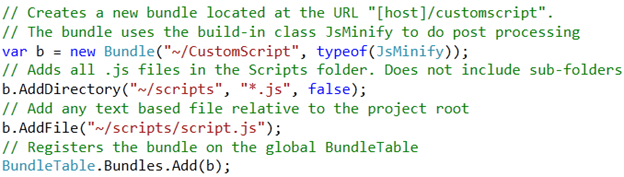
The above custom bundle can then be referenced anywhere within the application using the below <script> reference:
Custom Processing
You can also override the default CSS and JavaScript bundles to support your own custom processing of the bundled files (for example: custom minification rules, support for Saas, LESS or Coffeescript syntax, etc).
In the example below we are indicating that we want to replace the built-in minification transforms with a custom MyJsTransform and MyCssTransform class. They both subclass the CSS and JavaScript minifier respectively and can add extra functionality:

The end result of this extensibility is that you can plug-into the bundling/minification logic at a deep level and do some pretty cool things with it.
2 Minute Video of Bundling and Minification in Action
Mads Kristensen has a great
90 second video that shows off using the new Bundling and Minification feature. You can watch the 90 second video
here.
Summary
The new bundling and minification support within the next release of ASP.NET will make it easier to build fast web applications. It is really easy to use, and doesn’t require major changes to your existing dev workflow. It is also supports a rich extensibility API that enables you to customize it however you want.
You can easily take advantage of this new support within ASP.NET MVC, ASP.NET Web Forms and ASP.NET Web Pages based applications.
This blog post has been originally written by
ScottGu on Monday, November 28, 2011 1:58 AM I have published it as I think my followers will benefit from it.